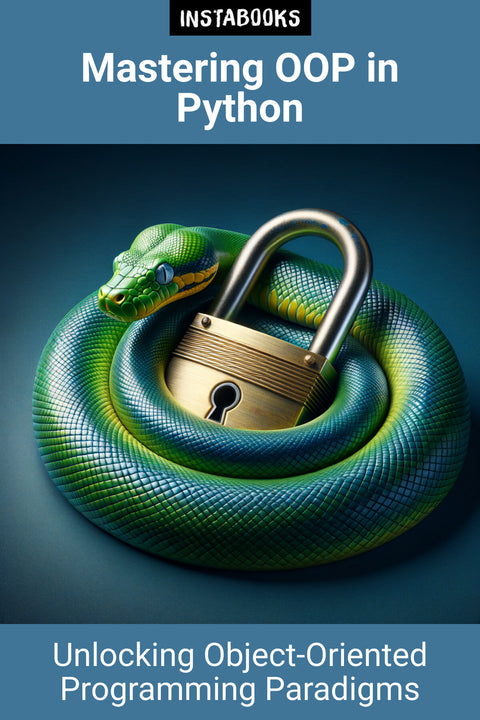
Instabooks AI (AI Author)
Mastering OOP in Python
Unlocking Object-Oriented Programming Paradigms
Premium AI Book (PDF/ePub) - 200+ pages
Table of Contents
1. The Pillars of OOP in Python- Understanding Classes and Objects
- The Power of Inheritance
- Interfaces and Polymorphism
2. Writing Reusable Code
- Leveraging Modules and Packages
- Design Patterns in Python
- Refactoring for Efficiency
3. Advanced OOP: Beyond the Basics
- Metaclasses and Attributes
- Managing Multiple Inheritance
- Magic Methods & Operator Overloading
4. Practical OOP: Projects and Solutions
- Building a Command-Line Application
- Developing a Web Service with Flask
- Creating a Game with Pygame
5. OOP Design Principles
- SOLID Principles Explained
- Applying the DRY Concept
- Balancing Cohesion and Coupling
6. Python Data Models
- Understanding the Data Model
- Customizing Object Behavior
- The Importance of __slots__
7. Exception Handling in Python
- Basics of Exceptions
- Designing Robust Code
- Custom Exception Types
8. Testing Your OOP Code
- Unit Testing Basics
- Test-Driven Development (TDD)
- Using Mocks and Stubs
9. OOP and Multithreading
- Threads vs. Processes
- Synchronizing Threads
- Threading in GUI Applications
10. Performance Optimization
- Profiling OOP Code
- Optimizing Memory Usage
- Speeding Up Python with Cython
11. Integrating with Other Languages
- Extending Python with C/C++
- Using Python with Java
- The Role of Python in Data Science
12. The Future of OOP in Python
- Keeping Up with Python Updates
- Python's Role in Modern Software
- Evolution of OOP Practices
How This Book Was Generated
This book is the result of our advanced AI text generator, meticulously crafted to deliver not just information but meaningful insights. By leveraging our AI book generator, cutting-edge models, and real-time research, we ensure each page reflects the most current and reliable knowledge. Our AI processes vast data with unmatched precision, producing over 200 pages of coherent, authoritative content. This isn’t just a collection of facts—it’s a thoughtfully crafted narrative, shaped by our technology, that engages the mind and resonates with the reader, offering a deep, trustworthy exploration of the subject.
Satisfaction Guaranteed: Try It Risk-Free
We invite you to try it out for yourself, backed by our no-questions-asked money-back guarantee. If you're not completely satisfied, we'll refund your purchase—no strings attached.